Kickstart Your IT Career with MindScripts Tech! 🚀 Expert-Led Training | 3 Days Free Demo | 100% Placement Assistance | Join 1500+ Company Tie-Ups Today! Ready to Succeed? Let’s Begin!
Mastering the Basics: 20 Essential Java Developer Interview Questions and Answers for Freshers
If you're gearing up for a Java Developer interview as a fresher, you're in the right place. In this post, we'll explore key questions that interviewers often ask candidates to assess their understanding of Java fundamentals. Whether you're a recent graduate or someone looking to kickstart your career in Java development, these questions and comprehensive answers will help you prepare and boost your confidence for the big day. Let's dive into the world of Java development and set you on the path to success!
MindScripts Tech
1/24/20244 min read
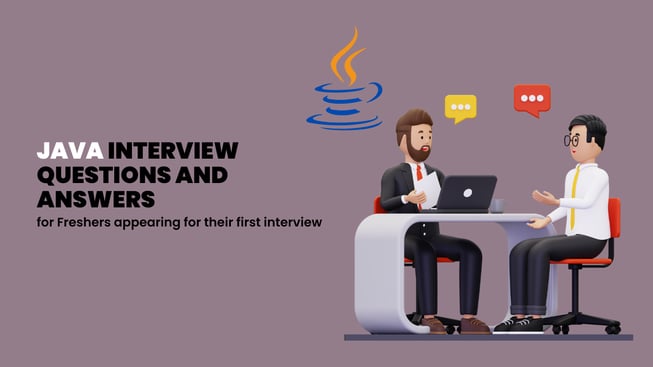
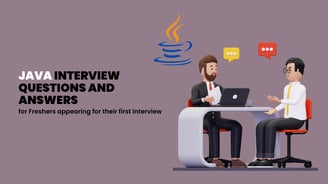
1. What is Java?
- Answer: Java is a high-level, object-oriented programming language developed by Sun Microsystems. It is designed to be platform-independent and follows the "Write Once, Run Anywhere" (WORA) principle.
2. What are the key features of Java?
- Answer: Key features of Java include:
- Object-oriented
- Platform-independent
- Simple and easy to learn
- Robust and secure
- Multithreading
- Distributed computing support through RMI (Remote Method Invocation)
- Dynamic and extensible
3. What is the difference between JDK, JRE, and JVM?
- Answer:
- JVM (Java Virtual Machine): It is an abstract machine that provides the runtime environment for Java bytecode to be executed.
- JRE (Java Runtime Environment): It includes the JVM, libraries, and other components required for running Java applications but does not include development tools.
- JDK (Java Development Kit): It includes the JRE along with development tools like compiler and debugger for Java application development.
4. Explain the main principles of object-oriented programming (OOP) and how Java supports them.
- Answer: OOP principles include encapsulation, inheritance, and polymorphism. Java supports these principles through class and interface definitions, access modifiers, and the ability to create and use objects.
5. What is the difference between abstract classes and interfaces?
- Answer: Abstract classes can have both abstract and concrete methods, while interfaces can only have abstract methods. A class can extend only one abstract class, but it can implement multiple interfaces.
6. Explain the concept of multithreading in Java.
- Answer: Multithreading is the concurrent execution of two or more threads. Threads in Java are lightweight processes that share the same memory space. Java provides the `Thread` class and the `Runnable` interface to implement multithreading.
7. What is the purpose of the `finalize` method?
- Answer: The `finalize` method is called by the garbage collector before an object is reclaimed. It allows the object to release any resources it holds before being garbage collected.
8. How does exception handling work in Java?
- Answer: Java uses a try-catch block to handle exceptions. Code that may throw an exception is placed in the try block, and the catch block contains the code to handle the exception. Additionally, there's a final block for code that should be executed regardless of whether an exception is thrown or not.
9. Explain the concept of the Java Collections Framework.
- Answer: The Java Collections Framework provides a set of classes and interfaces for handling and manipulating groups of objects. It includes interfaces like `List`, `Set`, and `Map`, along with their respective implementations like `ArrayList`, `HashSet`, and `HashMap`.
10. How does garbage collection work in Java?
- Answer: Garbage collection in Java is an automatic process where the JVM identifies and removes unreferenced objects to free up memory. The primary mechanism is the use of the garbage collector, which runs in the background and cleans up objects that are no longer reachable.
11. What is the difference between `String`, `StringBuilder`, and `StringBuffer` in Java?
- Answer: `String` is immutable, while `StringBuilder` and `StringBuffer` are mutable. `StringBuilder` is not thread-safe, whereas `StringBuffer` is thread-safe due to its synchronized methods. For most scenarios, `StringBuilder` is preferred for better performance.
12. Explain the concept of method overloading in Java.
- Answer: Method overloading allows a class to have multiple methods with the same name but different parameters. It is determined at compile time-based on the number or types of arguments. Overloaded methods must have a different number or type of parameters.
13. What is the `static` keyword in Java?
- Answer: The `static` keyword is used to declare members (variables and methods) that belong to the class rather than instances of the class. Static members are shared among all instances of a class and can be accessed using the class name.
14. How does Java support polymorphism?
- Answer: Polymorphism in Java is achieved through method overloading and method overriding. Method overloading allows multiple methods with the same name but different parameters, while method overriding occurs when a subclass provides a specific implementation for a method defined in its superclass.
15. Explain the concept of serialization in Java.
- Answer: Serialization is the process of converting an object into a byte stream to persist its state or transmit it over a network. In Java, the `Serializable` interface is implemented by classes to indicate that their objects can be serialized. The `ObjectOutputStream` and `ObjectInputStream` classes are used for serialization and deserialization.
16. What is the purpose of the `equals()` and `hashCode()` methods in Java?
- Answer: The `equals()` method is used to compare the content of objects for equality, while the `hashCode()` method returns a hash code value for an object. These methods are often overridden together to ensure consistency; if two objects are equal, their hash codes must be equal as well.
17. What is the significance of the `this` keyword in Java?
- Answer: The `this` keyword in Java refers to the current instance of the class. It is used to differentiate instance variables from local variables when they have the same name, and it can be used to invoke the current object's method.
18. How does Java handle memory management, and what is the role of the garbage collector?
- Answer: Java uses automatic memory management, and the garbage collector is responsible for reclaiming memory occupied by objects that are no longer reachable. The garbage collector identifies and removes unreferenced objects, freeing up memory for new objects.
19. What is the purpose of the `super` keyword in Java?
- Answer: The `super` keyword is used to refer to the superclass (parent class) of the current class. It can be used to call the superclass's methods, access its fields, or invoke its constructor. It is often used in method overriding to call the superclass implementation.
20. Explain the concept of design patterns in Java. Can you name a few design patterns?
- Answer: Design patterns are general reusable solutions to common problems encountered in software design. Examples of design patterns include Singleton, Factory, Observer, and MVC (Model-View-Controller). These patterns promote best practices in object-oriented design and architecture.
Remember to practice these questions and ensure a good understanding of the underlying concepts. Good luck with your interview!
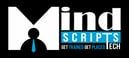

At MindScripts Tech, our mission is to empower individuals through cutting-edge IT education, bridging theory with practical skills. We cultivate innovation, nurture creativity, and prepare our students to thrive in the dynamic IT industry, fostering a globally competitive workforce.